Python
topguide
- 0
Python Installation
There are two ways to download and install Python
- Download Python from its official website. You have to manually install libraries.
- Download Anaconda. It comes with python and also popular python modules.
Useful commands:
pip list | To get the installed packages |
python – – version | To check the python version |
pip show pandas | To display Pandas Version |
print(pandas.__version__) | To display Pandas Version |
pandas.show_versions() | It comprises info about hosting OS, Pandas version, and versions of other installed packages |
pip install pandas | To upgrade the Pandas module |
pip install – – upgrade pandas – – user | To upgrade the Pandas module |
conda – – version | To display conda version |
conda env list | To display conda environment list |
conda activate base | To activate base conda environment |
conda deactivate | Deactivate all conda environments |
Python Datatypes
Type | Description | Sample Values | Create | Access Variable & its Value |
string | ordered, immutable, collection datatype | ‘a’, ‘london’, ‘lon123’ , ‘how are you’ | ‘a’ or “he’s” | Refer appendix below |
list | ordered, mutable, collection datatype list can have different datatype Accessing element in a list by referring its index | [‘a’, 1, 3.5, ‘Hello’] | list( ) or [ ] | e.g. var_lst[0] out: ‘a’ Refer appendix for more details |
tuple | ordered, immutable, collection datatype often used for objects belong together. can’t create a tuple with single value i.e. then it is called as string. To create a tuple with single value add comma at the end. e.g. (‘a’,) When passing multiple values to the variable then automatically it create a tuple. | ( ) – optional | ||
set | unordered, mutable, no duplicate | set( ) or { } | ||
dictionary | unordered, mutable, collection datatype key value pair mutable – value of the key can be overwrritten | dict_var={“name”:”Ravi”, “location”:”London”} or dict_var=dict(name=”Ravi”, location=”London”) Note: 2nd method don’t need double quotes and “=” symbol used instead of “:” | dict() or { : } | dict_var[“name”] If no key then we get key don’t exist error or dict_var.get(“name”) If no key nothing gets outputted and won’t cause any key error. |
Pandas Datatypes
Int64 | Int |
float64 | Float |
object | str or mixed (e.g. numeric and non numeric) |
boolean | True/False |
Appendix:
Accessing String variable sample:
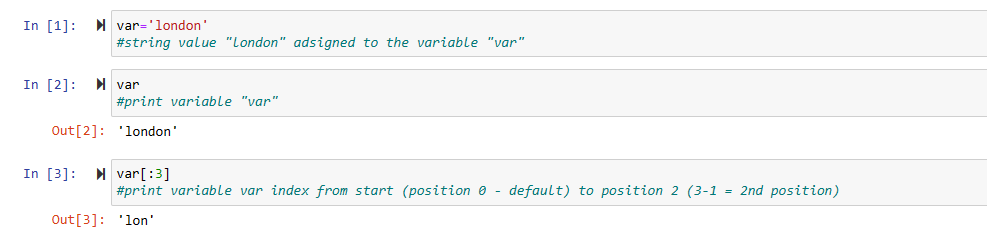
List and also Error handling
Note: Some of the topics listed to be updated later
Datatypes in python¶
- int
- string
- tuple
- list
- set
- dictionary
- boolean
List in python¶
- collection datatype
- allow duplciates
- ordered
- mutable
- list can accomodate different datatypes inside the list
Topics¶
- Create
- add items or remove items
- iterate list or accessing items inside the list
- list slicing
- list copy
- transform the items inside the list (list comp)
- destroy
- Use case
Create¶
In [2]:
# empty list creation - can add items to teh list at a later stage
lst=[]
In [5]:
# empty list creation - Another way
lst=list()
In [6]:
type(lst)
Out[6]:
list
In [7]:
len(lst)
Out[7]:
0
In [10]:
lst.append(10)
In [15]:
lst
Out[15]:
[10]
In [16]:
lst.append('rrr')
In [17]:
lst
Out[17]:
[10, 'rrr']
In [27]:
lst=[20,'ddd',300]
In [28]:
lst
Out[28]:
[20, 'ddd', 300]
Add Items to the list¶
In [29]:
#Append add valiues at the end
lst.append(10)
lst
Out[29]:
[20, 'ddd', 300, 10]
In [30]:
#To add values to the specific position -use "insert" instead of "append"
lst.insert(2,'hello')
lst
Out[30]:
[20, 'ddd', 'hello', 300, 10]
In [31]:
# remove the last element in a list
lst.pop()
lst
Out[31]:
[20, 'ddd', 'hello', 300]
Remove items to the list¶
In [32]:
# to remove an value from a list
lst.remove('ddd')
lst
# caution will get a value error if the given value is not present
Out[32]:
[20, 'hello', 300]
Error Handling¶
In [44]:
# we already deleted 'ddd' and lets try to issue the remove again and catch that error
lst=[20,300]
try:
lst.remove('ddd')
print(lst)
except ValueError as e:
print(e)
print("Error: given value is not there in the list")
except Exception as e:
print(e)
print("Some error occurred")
else: # if success
print(lst)
finally:
print('Program reaches to the finally block')
print("Bye")
list.remove(x): x not in list Error: given value is not there in the list Program reaches to the finally block Bye
In [43]:
lst=[20,300]
try:
lst.remove(300)
print(lst)
except ValueError as e:
print(e)
print("given value is not there in the list")
except Exception as e:
print(e)
print("Some error occurred")
else: # if success
print(lst)
finally:
print('Program reaches to the finally block')
print("Bye")
[20] [20] Program reaches to the finally block Bye
In [ ]: